Creating a scientific calculator using Python with a Tkinter GUI and a Flask API is an exciting project that covers multiple topics like GUI development, web APIs, and mathematical operations. The post provides step-by-step instructions for setting up the project with code examples.
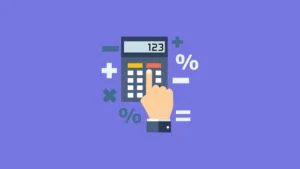
Table of Contents
Prerequisite:
- Install falsk restful : https://pypi.org/project/Flask-RESTful/
- Understanding of Rest API
- Understanding of Python 3.x
pip install Flask-RESTful
Step 1: Create Flask API
In the first step, you create a Flask API by writing code that handles POST requests with JSON data. The code extracts the operation, num1, and num2 from the request, performs the corresponding mathematical operation, and returns the result in JSON format.
Create a new Python file named calculator_api.py
and write the following code:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/calculate', methods=['POST'])
def calculate():
try:
data = request.json
operation = data['operation']
num1 = float(data['num1'])
num2 = float(data['num2'])
if operation == 'add':
result = num1 + num2
elif operation == 'subtract':
result = num1 - num2
elif operation == 'multiply':
result = num1 * num2
elif operation == 'divide':
if num2 == 0:
return jsonify({'error': 'Cannot divide by zero'})
result = num1 / num2
else:
return jsonify({'error': 'Invalid operation'})
return jsonify({'result': result})
except Exception as e:
return jsonify({'error': str(e)})
if __name__ == '__main__':
app.run(port=3000)
Step 2: Create Tkinter GUI
Next, you create a Tkinter GUI by writing code that creates an interface with input fields for num1, num2, and a dropdown menu for selecting the desired operation. There is also a button that triggers the calculation when clicked. The GUI code uses the requests library to send a POST request to the Flask API, passing the operation and numeric inputs. The response is then displayed in a label below.
Create another Python file named calculator_gui.py
and write the following code:
import tkinter as tk
from tkinter import ttk
import requests
def make_calculation():
num1 = float(entry1.get())
num2 = float(entry2.get())
operation = operation_var.get()
data = {'operation': operation, 'num1': num1, 'num2': num2}
response = requests.post('http://localhost:3000/calculate', json=data)
result = response.json()
if 'error' in result:
output_label.config(text=f"Error: {result['error']}")
else:
output_label.config(text=f"Result: {result['result']}")
# GUI
root = tk.Tk()
root.title('Scientific Calculator')
entry1 = ttk.Entry(root)
entry1.grid(row=0, column=1)
entry2 = ttk.Entry(root)
entry2.grid(row=1, column=1)
operation_var = tk.StringVar()
operation_var.set('add')
operations = ['add', 'subtract', 'multiply', 'divide']
operation_menu = ttk.OptionMenu(root, operation_var, *operations)
operation_menu.grid(row=2, column=1)
calculate_button = ttk.Button(root, text='Calculate', command=make_calculation)
calculate_button.grid(row=3, column=1)
output_label = ttk.Label(root, text='Result:')
output_label.grid(row=4, column=1)
root.mainloop()
Step 3: Run Both Programs
Finally, running both programs simultaneously to use the calculator. You start the Flask API by running the calculator_api.py
file and then run the calculator_gui.py
file to launch the Tkinter GUI. The GUI allows you to enter numbers and select an operation, and it displays the calculated result.
- Run
calculator_api.py
to start the Flask API. - Run
calculator_gui.py
to start the Tkinter GUI.
To use the calculator, enter numbers in the Tkinter GUI and select the operation you want to perform. Then, click “Calculate”. The result should appear below.
FAQ
What is Tkinter?
Tkinter is a standard Python library for creating graphical user interfaces (GUIs). It provides a set of tools and widgets for building desktop applications.
Is Tkinter included with Python?
Yes, Tkinter is included with most Python installations, so you don’t need to install it separately.
How do I create a basic window in Tkinter?
You can create a basic window (or GUI) in Tkinter using the Tk()
constructor. For example:python import tkinter as tk root = tk.Tk() root.mainloop()
What are widgets in Tkinter?
Widgets are GUI elements like buttons, labels, text entry fields, etc., that you can place in a Tkinter window to interact with users.
What is Flask?
Flask is a micro web framework for Python used to develop web applications, including RESTful APIs. It is known for its simplicity and flexibility.
How do I create a basic Flask API?
To create a basic Flask API, you need to define routes and functions that handle HTTP requests. You can use decorators like @app.route('/')
to specify the URL route.
What is RESTful API and how does Flask support it?
A RESTful API follows the principles of Representational State Transfer (REST). Flask supports RESTful API development by allowing you to define routes for different HTTP methods (GET, POST, PUT, DELETE) and use them to perform CRUD operations on resources.
Is Flask suitable for building large-scale APIs?
Flask can be used to build APIs of various sizes, but for large-scale applications, you may consider using additional libraries and structuring your project appropriately.