Here are the most popular interview questions and answers on Python’s NumPy library.
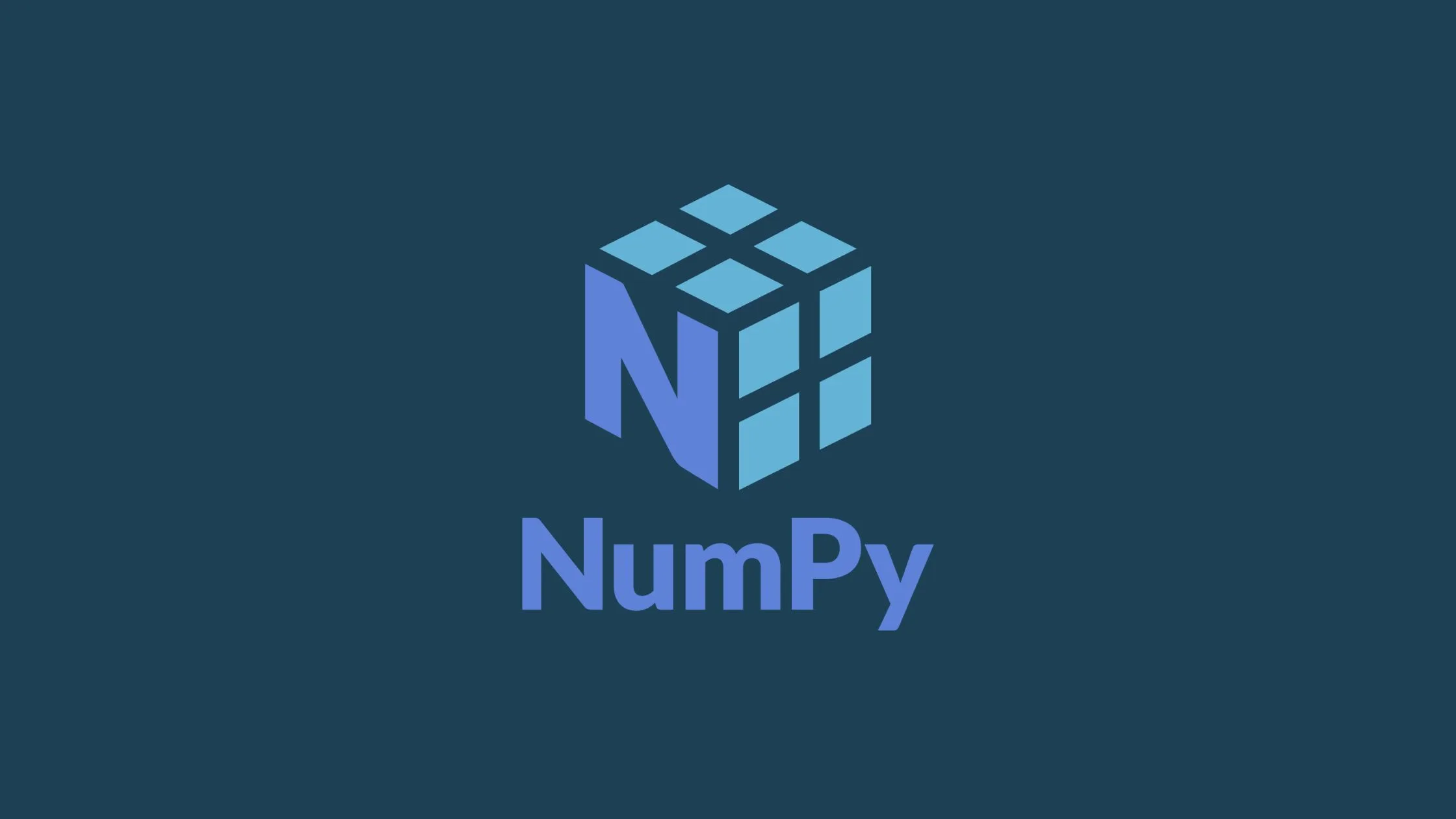
1. What is NumPy library?
Answer: NumPy stands for Numerical Python. It is a library in Python that provides support for large, multi-dimensional arrays and matrices, along with mathematical functions to operate on them.
2. How do you install NumPy library?
Answer: You can install NumPy using pip by running the command pip install numpy
.
3. What are NumPy arrays?
Answer: NumPy arrays are n-dimensional array objects that serve as containers for homogenous data types. They are more efficient and faster than Python’s native lists for numerical operations.
4. How do you create a NumPy array?
Answer: You can create a NumPy array using the numpy.array()
function, like so:
import numpy as np
arr = np.array([1, 2, 3, 4])
5. What is the difference between a Python list and a NumPy array?
Answer: Python lists can contain elements of different data types, while NumPy arrays are homogenous. NumPy arrays are more efficient in terms of memory and speed for numerical computations.
6. What is a NumPy ndarray?
Answer: The term “ndarray” stands for “n-dimensional array.” It is the primary object that NumPy uses to perform various operations.
7. What is the use of the shape
attribute in NumPy?
Answer: The shape
attribute returns a tuple representing the dimensions (shape) of the array.
8. How can you change the shape of a NumPy array?
Answer: You can use the reshape()
function to change the shape of an array.
9. What does the numpy.zeros()
function do?
Answer: It creates an array filled with zeros with the specified shape.
10. What does the numpy.ones()
function do?
Answer: It creates an array filled with ones with the specified shape.
11. What is slicing in NumPy library library?
Answer: Slicing in NumPy refers to extracting a portion of an array using indices.
12. How do you perform element-wise operations in NumPy?
Answer: Element-wise operations can be performed using the arithmetic operators like +
, -
, *
, and /
.
13. What is broadcasting in NumPy?
Answer: Broadcasting is a feature that allows NumPy to perform arithmetic operations on arrays with different shapes, by replicating the smaller array to match the shape of the larger array.
14. How do you find the minimum and maximum values in a NumPy array?
Answer: You can use the min()
and max()
functions to find the minimum and maximum values.
15. What are NumPy’s mathematical functions like sin
, cos
, and tan
used for?
Answer: These functions provide support for fast mathematical operations on arrays and are useful in scientific computing.
16. How do you concatenate two NumPy arrays?
Answer: You can use numpy.concatenate()
or numpy.hstack()
and numpy.vstack()
to concatenate arrays.
17. What is the dot product in NumPy?
Answer: The dot product is a mathematical operation that takes two arrays and returns a single value or an array.
18. What is a NumPy slice?
Answer: A NumPy slice is a subset of an array generated by specifying indices.
19. How do you convert a 1D array into a 2D array?
Answer: You can use the reshape()
method to convert a 1D array into a 2D array.
20. How do you perform matrix multiplication in NumPy?
Answer: You can use the dot()
function or the @
operator to perform matrix multiplication.
21. What is the purpose of the numpy.linspace()
function?
Answer: It generates an array of evenly spaced values over a specified range.
22. What are the uses of the axis
parameter in NumPy functions?
Answer: The axis
parameter specifies along which axis the operation should take place.
23. How do you invert a matrix in NumPy library?
Answer: You can use the numpy.linalg.inv()
function to invert a matrix.
24. What does the numpy.random
module do?
Answer: The numpy.random
module provides functions to generate random numbers and random samples.
25. What is the purpose of numpy.empty()
?
Answer: The numpy.empty()
function creates an uninitialized array of specified shape and dtype.
26. How do you compute the determinant of a matrix in NumPy?
Answer: You can use the numpy.linalg.det()
function to compute the determinant.
27. What is the NumPy equivalent of Python’s range function?
Answer: The NumPy equivalent of Python’s range function is numpy.arange()
.
28. What is numpy.transpose()
used for?
Answer: It is used for reversing or permuting the axes of an array or matrix.
29. How do you calculate the mean, median, and standard deviation using NumPy?
Answer: You can use numpy.mean()
, numpy.median()
, and numpy.std()
functions respectively to calculate these statistical measures.
30. How do you save and load NumPy objects?
Answer: You can use numpy.save()
to save an array to a binary file and numpy.load()
to load an array from a binary file.