Sets are a unique data structure in Python, allowing you to store an unordered collection of distinct elements. If you’re coming from a mathematical background, you’ll find that Python sets behave much like mathematical sets. This guide will explore the Python’s set data type, detailing how to create and use sets with various examples.
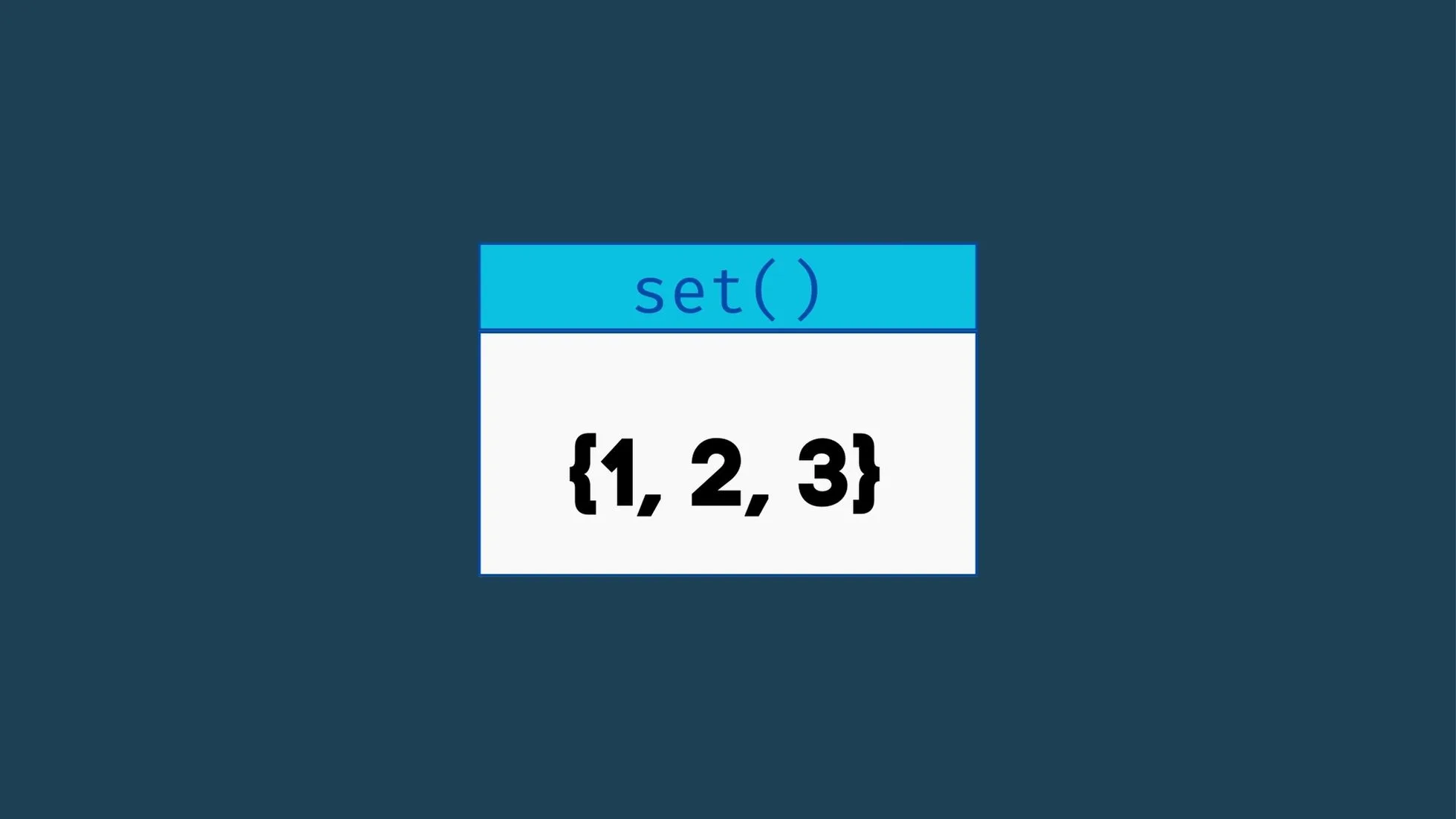
Table of Contents
- What is a Set?
- Creating a Set
- Adding Elements to a Set
- Removing Elements from a Set
- Set Operations
- Set Comprehensions
- Frequently Asked Questions on Python Set Data Type
- Conclusion
- Tutorials
- Quiz
What is a Set?
A set is an unordered collection of unique items. This means that a set cannot have duplicate values, and the order of elements is not maintained. Sets are mutable, meaning you can add and remove elements after the set has been created.
Creating a Set
You can create a set in Python using curly braces {}
or the set()
function.
1. Using Curly Braces
my_set = {1, 2, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
2. Using the set()
Function
my_set = set([1, 2, 3, 4])
print(my_set) # Output: {1, 2, 3, 4}
Adding Elements to a Set
You can add elements to a set using the add()
method.
my_set = {1, 2, 3}
my_set.add(4)
print(my_set) # Output: {1, 2, 3, 4}
Removing Elements from a Set
You can remove elements from a set using the remove()
or discard()
method.
my_set.remove(2)
print(my_set) # Output: {1, 3, 4}
my_set.discard(3)
print(my_set) # Output: {1, 4}
Set Operations
Sets provide various operations that allow you to perform mathematical set functions.
Union
The union of two sets is a set containing all elements from both sets. You can use the union()
method or the |
operator.
A = {1, 2, 3}
B = {3, 4, 5}
union_set = A.union(B)
print(union_set) # Output: {1, 2, 3, 4, 5}
Intersection
The intersection of two sets is a set containing only the elements that are common to both sets. You can use the intersection()
method or the &
operator.
intersection_set = A.intersection(B)
print(intersection_set) # Output: {3}
Difference
The difference of two sets is a set containing elements in the first set but not in the second set. You can use the difference()
method or the -
operator.
difference_set = A.difference(B)
print(difference_set) # Output: {1, 2}
Set Comprehensions
Just like lists and dictionaries, sets also support comprehensions.
squared_set = {x**2 for x in {1, 2, 3, 4}}
print(squared_set) # Output: {16, 1, 4, 9}
Frequently Asked Questions on Python Set Data Type
What is a set in Python?
A set is an unordered collection of unique elements in Python. Sets are mutable, meaning you can add or remove elements after the set has been created. They are defined by enclosing the elements in curly braces {}
or by using the built-in set()
constructor.
my_set = {1, 2, 3}
another_set = set([4, 5, 6])
How do you create a set?
You can create a set by enclosing elements in curly braces or using the set()
constructor.
# Using curly braces
my_set = {1, 2, 3}
# Using set() constructor
another_set = set([1, 2, 3])
Are sets ordered in Python?
No, sets are unordered collections. This means that the elements in a set have no index, and there is no guarantee of order.
Can a set contain duplicate elements?
No, sets cannot contain duplicate elements. Adding a duplicate element to a set will have no effect.
my_set = {1, 2, 3, 3, 4}
print(my_set) # Output: {1, 2, 3, 4}
How do you add elements to a set?
You can add elements to a set using the add()
method.
my_set = {1, 2, 3}
my_set.add(4) # Adds 4 to the set
How do you remove elements from a set?
You can remove elements using the remove()
or discard()
methods.
remove()
raises an error if the element is not found.discard()
does nothing if the element is not found.
my_set.remove(3) # Removes 3 from the set
my_set.discard(5) # Does nothing if 5 is not found
How do you perform set operations?
Python sets support operations like union, intersection, and difference.
A = {1, 2, 3}
B = {3, 4, 5}
# Union
print(A | B) # Output: {1, 2, 3, 4, 5}
# Intersection
print(A & B) # Output: {3}
# Difference
print(A - B) # Output: {1, 2}
How do you check if an element exists in a set?
You can use the in
keyword to check for membership.
my_set = {1, 2, 3}
print(1 in my_set) # Output: True
Can sets contain elements of multiple data types?
Yes, sets can contain elements of different data types, but the elements themselves must be immutable (e.g., numbers, strings, tuples).
my_set = {1, "apple", (2, 3)}
Can a set contain another set?
No, sets cannot contain other sets because sets are mutable. However, you can use a frozenset if you need a set-like structure that can be nested within another set.
nested_set = {1, 2, frozenset({3, 4})}
I hope this FAQ helps you better understand Python sets! Feel free to ask more questions.
Conclusion
Sets in Python are a powerful and flexible data structure, perfect for handling collections of unique elements. With various built-in methods for manipulation and mathematical operations, sets are an invaluable tool for any Python programmer.
Whether you’re handling data analysis or building complex algorithms, understanding sets will undoubtedly enhance your coding capabilities.