Logical operators are a fundamental part of programming, allowing us to make decisions based on certain conditions. In Python, logical operators are used to combine conditional statements. This guide will explore the three main logical operators in Python: and
, or
, and not
.
Table of Contents
- The and Operator
- The or Operator
- The not Operator
- Combining Logical Operators
- Summary
- FAQ
- Conclusion
- Quizzes
- Tutorial
The and
Operator
The and
operator returns True
if both the left and right expressions are true. If either expression is false, the entire statement evaluates to False
.
Example:
x = 10
y = 5
# Both conditions are true, so the result is True
result = x > y and x == 10
print(result) # Output: True
# One condition is false, so the result is False
result = x < y and x == 10
print(result) # Output: False
The or
Operator
The or
operator returns True
if at least one of the expressions is true. If both expressions are false, the entire statement evaluates to False
.
Example:
x = 10
y = 5
# One condition is true, so the result is True
result = x < y or x == 10
print(result) # Output: True
# Both conditions are false, so the result is False
result = x < y or x == 0
print(result) # Output: False
The not
Operator
The not
operator returns the opposite of the expression’s truth value. If the expression is true, not
returns False
, and if the expression is false, not
returns True
.
Example:
x = 10
# The condition is true, so the result is False
result = not x == 10
print(result) # Output: False
# The condition is false, so the result is True
result = not x < 5
print(result) # Output: True
Combining Logical Operators
You can combine these logical operators to create more complex conditions.
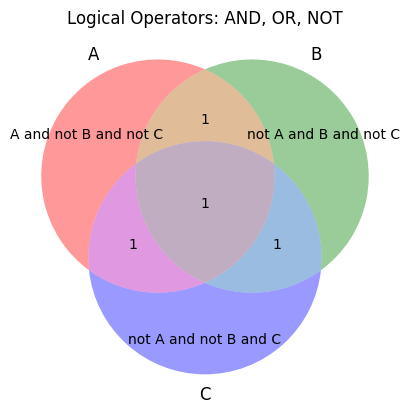
Example:
x = 10
y = 5
z = 20
# Combining and, or, and not
result = (x > y and z > x) or not (y == z)
print(result) # Output: True
Summary
Logical operators in Python are essential for controlling the flow of your program based on specific conditions. Understanding how to use the and
, or
, and not
operators allows you to create more complex and dynamic code.
Remember to use parentheses when combining multiple logical operators to ensure the correct order of operations.
FAQ
1. What are Logical Operators in Python?
Answer:
Logical operators are used to combine conditional statements. Python supports three types of logical operators:
and
: Evaluates to True if both conditions are true.or
: Evaluates to True if at least one condition is true.not
: Inverts the boolean value of the condition it precedes.
x = 10
y = 20
# Using 'and'
if x > 5 and y > 15:
print("Both conditions are true.")
# Using 'or'
if x > 5 or y < 30:
print("At least one condition is true.")
# Using 'not'
if not x < 5:
print("x is not less than 5.")
2. What is Short-Circuiting?
Answer:
Short-circuiting refers to the behavior where the evaluation of a logical expression stops as soon as the outcome is determined. For example, in an or
operation, if the first condition is True, Python will not evaluate the second condition.
if True or my_function():
print("True, so my_function() is not called.")
3. Can Logical Operators be Chained?
Answer:
Yes, logical operators can be chained together to form more complex conditions.
if x > 5 and y > 15 and z < 25:
print("All conditions are true.")
4. Can Logical Operators Work with Non-Boolean Values?
Answer:
Yes, logical operators can work with non-Boolean values. In Python, non-Boolean values are evaluated as True
or False
based on their truthiness.
if "hello" and [1, 2, 3]:
print("Both are truthy values.")
Here, the string “hello” and the list [1, 2, 3]
are both truthy, so the and
operator evaluates to True
.
5. How is the not
Operator Used?
Answer:
The not
operator is used to invert the truth value of a condition.
if not False:
print("This will execute.")
In this example, not False
is equivalent to True
, so the print statement will execute.
Conclusion
Understanding Python’s logical operators is vital for controlling the flow of your programs. These operators allow you to create complex conditions efficiently and elegantly. Hopefully, this FAQ has clarified any questions you may have had. Keep practicing to become more proficient at using logical operators in Python!