Python, a popular high-level programming language, boasts of its easy-to-understand syntax and wide range of data types. Among these data types, python list data type stands out due to its versatility. This blog post will delve deep into Python list, exploring their features, methods, and potential use cases.
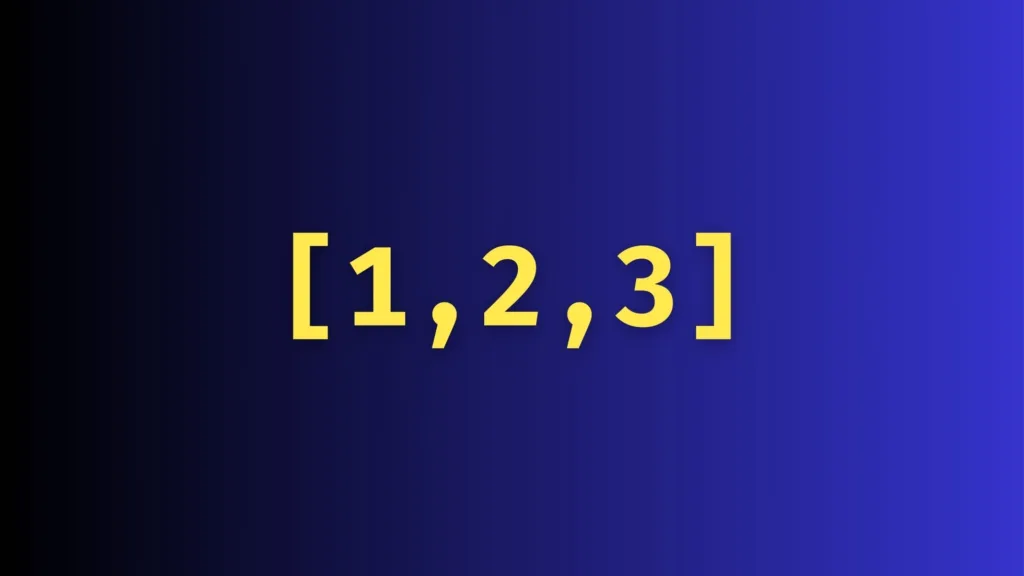
Table of Contents
- What is a Python List?
- How to Create a Python List?
- Accessing List Elements
- Modifying Lists
- Common List Methods
- Looping Through a List
- FAQs about Python List:
- Tutorial:
What is a Python List?
A list in Python is an ordered collection of items. These items can be of any type and can even be of mixed types. The items in a list are mutable, which means they can be changed after the list is created.
Example:
fruits = ['apple', 'banana', 'cherry']
print(fruits)
Output:
['apple', 'banana', 'cherry']
How to Create a Python List?
There are several ways to create lists in Python:
- Using square brackets
[]
- Using the list constructor
list()
Example:
# Using square brackets
fruits = ['apple', 'banana', 'cherry']
# Using list constructor
numbers = list((1, 2, 3, 4))
print(fruits)
print(numbers)
Output:
['apple', 'banana', 'cherry']
[1, 2, 3, 4]
Accessing List Elements
You can access the list items by referring to the index number. Remember that indexing in Python starts from 0.
Example:
fruits = ['apple', 'banana', 'cherry']
print(fruits[1]) # This will print 'banana'
Modifying Lists
Lists are mutable, which means you can change, add, or remove items after the list is created.
Example:
fruits = ['apple', 'banana', 'cherry']
fruits[1] = 'blueberry'
print(fruits) # ['apple', 'blueberry', 'cherry']
Common List Methods
append()
: Adds an item to the end of the list.extend()
: Adds multiple items to the list.insert()
: Adds an item at the specified position.remove()
: Removes the first occurrence of the item.pop()
: Removes the item at the specified position
Example:
fruits = ['apple', 'banana', 'cherry']
fruits.append('orange')
fruits.extend(['grape', 'kiwi'])
fruits.insert(2, 'mango')
fruits.remove('banana')
print(fruits.pop(2))
print(fruits)
Python lists come with a variety of built-in methods. Here’s a rundown of all the methods, along with examples for each:
1. append()
Adds an element at the end of the list.
Example:
fruits = ['apple', 'banana']
fruits.append('cherry')
print(fruits) # ['apple', 'banana', 'cherry']
2. clear()
Removes all the elements from the list.
Example:
fruits = ['apple', 'banana', 'cherry']
fruits.clear()
print(fruits) # []
3. copy()
Returns a copy of the list.
Example:
fruits = ['apple', 'banana', 'cherry']
new_list = fruits.copy()
print(new_list) # ['apple', 'banana', 'cherry']
4. count()
Returns the number of elements with the specified value.
Example:
fruits = ['apple', 'banana', 'cherry', 'apple']
print(fruits.count('apple')) # 2
5. extend()
Add the elements of a list (or any iterable), to the end of the current list.
Example:
fruits1 = ['apple', 'banana']
fruits2 = ['cherry', 'date']
fruits1.extend(fruits2)
print(fruits1) # ['apple', 'banana', 'cherry', 'date']
6. index()
Returns the index of the first element with the specified value.
Example:
fruits = ['apple', 'banana', 'cherry']
print(fruits.index('banana')) # 1
7. insert()
Adds an element at the specified position.
Example:
fruits = ['apple', 'cherry']
fruits.insert(1, 'banana')
print(fruits) # ['apple', 'banana', 'cherry']
8. pop()
Removes the element at the specified position.
Example:
fruits = ['apple', 'banana', 'cherry']
fruits.pop(1)
print(fruits) # ['apple', 'cherry']
9. remove()
Removes the first item with the specified value.
Example:
fruits = ['apple', 'banana', 'cherry']
fruits.remove('banana')
print(fruits) # ['apple', 'cherry']
10. reverse()
Reverses the order of the list.
Example:
fruits = ['apple', 'banana', 'cherry']
fruits.reverse()
print(fruits) # ['cherry', 'banana', 'apple']
11. sort()
Sorts the list.
Example:
fruits = ['cherry', 'apple', 'banana']
fruits.sort()
print(fruits) # ['apple', 'banana', 'cherry']
Looping Through a List
You can loop through a list using a for loop.
Example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
FAQs about Python List:
- How do you add an item to a list?
- Answer: There are several methods to add items to a list. The
append()
method adds an item to the end of the list, and theinsert()
method adds an item at a specified position.python fruits = ["apple", "banana"] fruits.append("cherry") # ["apple", "banana", "cherry"] fruits.insert(1, "orange") # ["apple", "orange", "banana", "cherry"]
2. How can you remove an item from a list?
- Answer: There are multiple methods to remove items. The
remove()
method removes the first occurrence of a specified item, thepop()
method removes the item at a specified position (or the last item if no index is provided), and thedel
statement removes the item at a specified index or a slice of items.python fruits = ["apple", "orange", "banana", "cherry"] fruits.remove("orange") # ["apple", "banana", "cherry"] fruits.pop(1) # ["apple", "cherry"] del fruits[0] # ["cherry"]
3. How do you find the index of an item in a list?
- Answer: You can use the
index()
method to find the position of the first occurrence of a specified value. If the item is not in the list, it will raise aValueError
.python numbers = [1, 2, 3, 4, 5] index_of_three = numbers.index(3) # Returns 2
4. Can a list contain multiple data types?
- Answer: Yes, a list can contain items of different data types, including numbers, strings, and other lists (nested lists).
python mixed_list = [1, "apple", [3, 4, 5], 2.5]
Lists are a fundamental and versatile data structure in Python, and there’s a lot more to explore beyond these FAQs!