In the realm of programming, data types form the building blocks of any language. One of the most fundamental data types in Python is the Python integers data type. Integers allow us to work with whole numbers, enabling a wide range of applications from basic arithmetic to more complex mathematical operations. In this post, we’ll delve into the world of Python integers, exploring their characteristics and providing 10 illustrative coding examples to showcase their versatility.
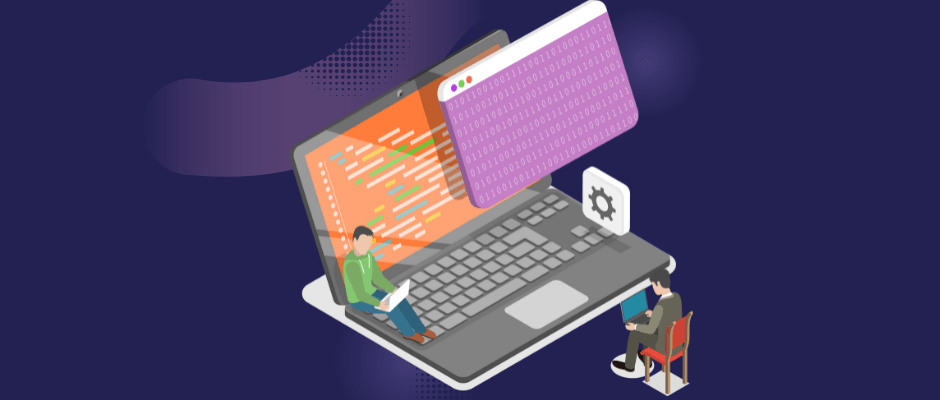
Table of Contents
- Understanding Python Integers
- Coding Examples for Python Integers
- Tutorial:
- Conclusion
- Frequently Asked Questions (FAQ) for Python Integers
- What is an integer data type in Python?
- How do I declare an integer in Python?
- What is the range of integers in Python?
- How do you perform arithmetic operations on integers?
- What is integer division?
- What is the modulo operator?
- Is there a type conversion function for integers?
- How do I check the data type of an integer?
- What are bitwise operators?
- How are integers stored in memory?
Understanding Python Integers
An integer is a whole number without a decimal point. It can be positive, negative, or zero. Python’s integer data type (int
) supports both signed and unsigned integers, making it suitable for a diverse range of numerical tasks.
Coding Examples for Python Integers
Let’s jump right into coding examples that highlight the usage and capabilities of Python integers:
1. Basic Arithmetic Operations
# Addition
sum_result = 5 + 3
# Subtraction
difference = 10 - 7
# Multiplication
product = 4 * 6
# Division
quotient = 15 / 3
# Floor Division
remainder = 17 // 5
# Modulo (Remainder)
modulo_result = 17 % 5
# Exponentiation
power_result = 2 ** 4
2. Negative Integers
negative_value = -10
3. Large Integers
Python’s integer support is not limited by the size of hardware memory:
large_int = 123456789012345678901234567890
4. Type Conversion
Convert integers to strings and vice versa:
int_to_str = str(25)
str_to_int = int("42")
5. Integer Operations with Other Data Types
# Integer and float
float_result = 10 + 5.0
# Integer and boolean
bool_result = 7 + True # True is treated as 1
6. Mixed Arithmetic
mixed_result = 5 * 2 + 3 // 2
7. Integer Division Behavior
div_result = 5 / 2 # Returns a floating-point result (2.5)
int_div_result = 5 // 2 # Returns an integer result (2)
8. Using Parentheses
result = (10 + 2) * 3
9. Increment and Decrement
Python doesn’t have a specific increment or decrement operator like some other languages, but you can achieve the same effect using arithmetic:
count = 0
count += 1 # Increment
count -= 1 # Decrement
10. Bases and Conversions
Python allows integers to be represented in different bases:
binary_num = 0b1010 # Binary
octal_num = 0o16 # Octal
hex_num = 0xA # Hexadecimal
Tutorial:
Tutorial for Python Integer Data Type
Conclusion
Python’s integer data type provides the foundation for numerical computations and operations in a wide array of programming scenarios. Its flexibility, support for basic arithmetic, and compatibility with other data types make it an essential tool for any programmer. Whether you’re calculating financial data, working on mathematical algorithms, or simply performing day-to-day calculations, Python integers are there to serve your needs. By mastering the intricacies of integers, you’re setting yourself up for success in the world of programming.
Frequently Asked Questions (FAQ) for Python Integers
What is an integer data type in Python?
In Python, an integer is one of the built-in data types used to represent whole numbers (both positive and negative) without a fractional component. It is denoted by the keyword int
.
How do I declare an integer in Python?
You don’t need to explicitly declare the data type in Python. When you assign a whole number to a variable, Python automatically treats it as an integer.
x = 10 # x is an integer
What is the range of integers in Python?
Python 3 has arbitrary-precision integers, which means they can grow as large as your computer’s memory allows. There is no maximum or minimum limit.
How do you perform arithmetic operations on integers?
You can perform arithmetic operations like addition, subtraction, multiplication, and division on integers using the respective operators (+
, -
, *
, /
).
a = 5
b = 2
addition = a + b # 7
subtraction = a - b # 3
multiplication = a * b # 10
division = a / b # 2.5
What is integer division?
Integer division is performed using the //
operator. The result is an integer obtained by truncating the decimal part.
result = 5 // 2 # Result is 2
What is the modulo operator?
The modulo operator %
returns the remainder of the division of the left-hand operand by the right-hand operand.
remainder = 10 % 3 # Result is 1
Is there a type conversion function for integers?
Yes, you can use the int()
function to convert other data types to integers.
x = int(5.5) # x becomes 5
y = int("10") # y becomes 10
How do I check the data type of an integer?
You can use the type()
function to check the data type of a variable.
x = 10
print(type(x)) # Output: <class 'int'>
What are bitwise operators?
Bitwise operators (&
, |
, ^
, ~
, <<
, >>
) perform operations on integers at the bit-level.
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a & b # Result is 1 (Binary: 0001)
How are integers stored in memory?
In Python, integers are objects. The amount of memory consumed can vary depending on the implementation and the size of the integer. You can use sys.getsizeof()
to get the size of an integer in bytes.
import sys
print(sys.getsizeof(10)) # Output may vary