Dictionaries are a cornerstone of Python programming, offering a powerful way to associate keys with values. Unlike lists and tuples, dictionaries store data in key-value pairs, making them highly flexible and efficient for various applications. This guide will delve into the creation and utilization of dictionaries in Python.
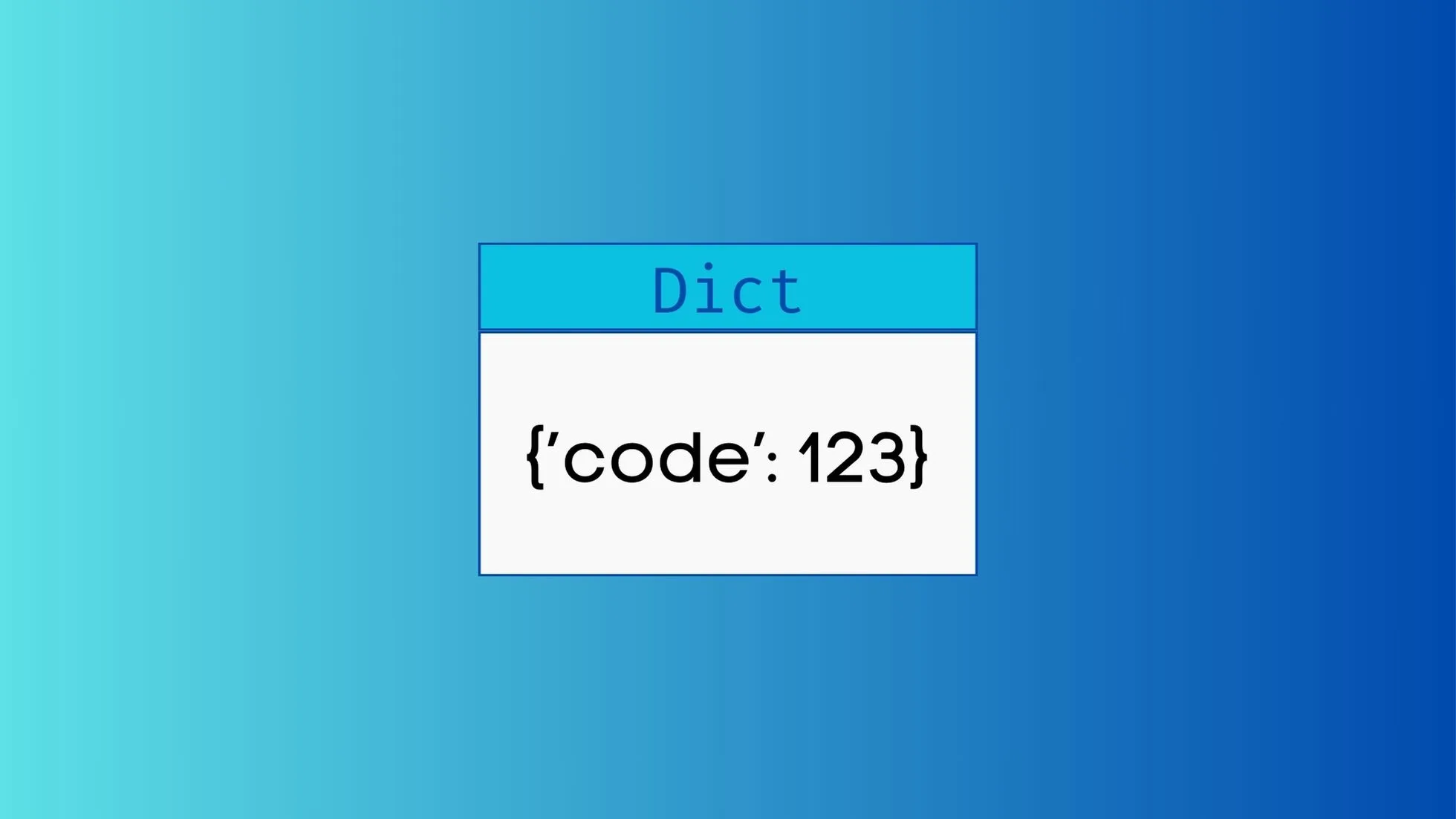
Table of Contents
- What is a Dictionary?
- Creating Dictionaries
- Accessing Values
- Modifying Dictionaries
- Iterating Through a Dictionary
- Dictionary Comprehensions
- FAQ for Python Dictionary Data Type:
- Conclusion
- Code Examples
- Tutorial
What is a Dictionary?
A dictionary in Python is an unordered collection of key-value pairs. The keys must be immutable (e.g., strings, numbers, tuples) and unique within the dictionary, while the values can be of any type.
Creating Dictionaries
1. Using Curly Braces {}
You can create a dictionary by enclosing key-value pairs in curly braces, separated by colons.
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
2. Using the dict()
Constructor
Alternatively, you can use the dict()
function.
my_dict = dict(name="Alice", age=30, city="New York")
Accessing Values
You can access a value in a dictionary by referring to its key.
name = my_dict["name"]
print(name) # Output: Alice
Modifying Dictionaries
Adding or Updating Key-Value Pairs
my_dict["country"] = "USA" # Adds a new key-value pair
my_dict["age"] = 31 # Updates the value of an existing key
Removing Key-Value Pairs
del my_dict["age"] # Removes the key-value pair with key "age"
Iterating Through a Dictionary
Iterating Through Keys
for key in my_dict:
print(key) # Prints all the keys
Iterating Through Values
for value in my_dict.values():
print(value) # Prints all the values
Iterating Through Key-Value Pairs
for key, value in my_dict.items():
print(key, value) # Prints all key-value pairs
Dictionary Comprehensions
You can use dictionary comprehensions to create dictionaries from existing iterables.
squares = {x: x**2 for x in range(1, 6)}
print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
FAQ for Python Dictionary Data Type:
What is a Dictionary in Python
A Dictionary in Python is an unordered, mutable collection of key-value pairs.
How do you create a Dictionary?
You can create a dictionary using curly braces {}
or the built-in dict()
function.
# Using curly braces
my_dict = {'key1': 'value1', 'key2': 'value2'}
# Using dict() function
my_dict = dict(key1='value1', key2='value2')
How do you access values in a Dictionary?
To access a value, you can use the key in square brackets.
value = my_dict['key1']
Alternatively, you can use the get()
method to access values, which returns None
if the key is not found.
value = my_dict.get('key1')
How to update or add key-value pairs?
You can update a value by assigning a new value to an existing key.
my_dict['key1'] = 'new_value1'
To add a new key-value pair, use a new key and assign a value to it.
my_dict['key3'] = 'value3'
How to delete key-value pairs?
You can delete a key-value pair using the del
keyword or the pop()
method.
# Using del keyword
del my_dict['key1']
# Using pop method
my_dict.pop('key1', None)
How to loop through a Dictionary?
You can loop through keys, values, or key-value pairs using keys()
, values()
, or items()
methods, respectively.
# Loop through keys
for key in my_dict.keys():
print(key)
# Loop through values
for value in my_dict.values():
print(value)
# Loop through key-value pairs
for key, value in my_dict.items():
print(key, value)
Can a Dictionary contain another Dictionary?
Yes, a dictionary can contain another dictionary. This is known as a nested dictionary.
nested_dict = {'outer_key': {'inner_key': 'inner_value'}}
Are Dictionary keys case-sensitive?
Yes, dictionary keys are case-sensitiveCan I use a list as a Dictionary key
Conclusion
Dictionaries in Python are an incredibly versatile and efficient data structure. Whether you’re storing configurations, mapping relationships, or caching results, dictionaries provide an intuitive way to handle key-value data.
By mastering the creation, access, modification, and iteration of dictionaries, you unlock a vital tool in your Python programming toolkit.