Introduction
Debugging is the process of identifying and fixing errors, also known as bugs, in your code. These errors can lead to unexpected behavior, crashes, or incorrect results in your programs. Python provides several tools and techniques to help you effectively debug your code and ensure its reliability. In this article we will understand the concepts of Debugging in Python
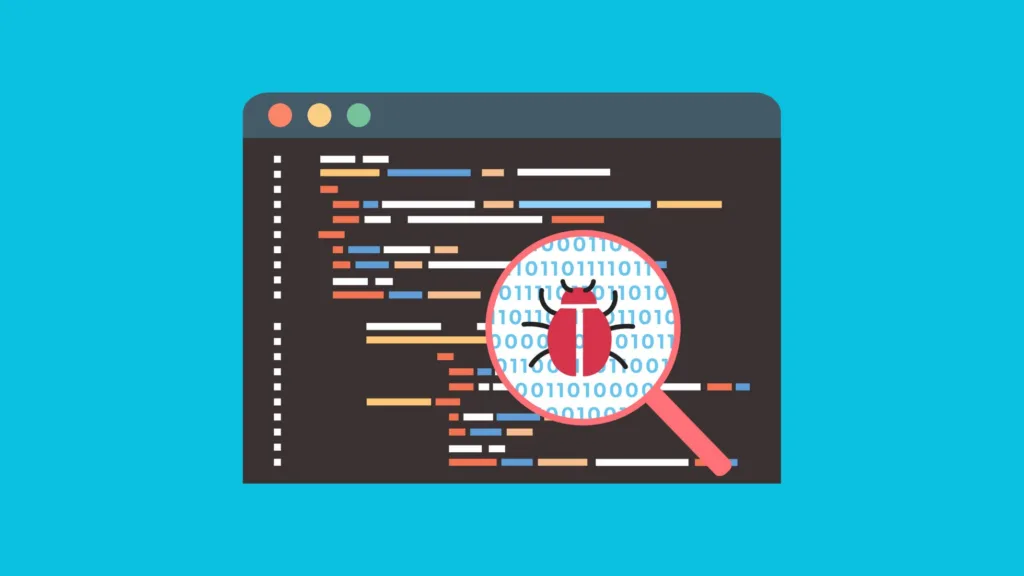
Benefits of Effective Debugging
- Saves Time: Debugging helps you locate and fix issues quickly, reducing the time spent searching for errors.
- Improved Code Quality: Fixing bugs improves the overall quality and reliability of your codebase.
- Enhanced Learning: Debugging provides insights into how code works and helps you learn from your mistakes.
- Effective Problem Solving: Debugging enhances your problem-solving skills by requiring careful analysis and logical thinking.
Common Debugging Concepts
1. Print Statements
Using print
statements to display variable values and intermediate results can help you understand how your code is executing.
def calculate_average(numbers):
total = sum(numbers)
count = len(numbers)
average = total / count
print("Total:", total, "Count:", count, "Average:", average)
return average
2. Using Breakpoints
IDEs like PyCharm and Visual Studio Code allow you to set breakpoints in your code, pausing execution at a specific line to inspect variables and step through the code.
3. Exception Handling
Wrap suspect code with try-except blocks to catch and handle exceptions gracefully.
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero.")
4. Assertion Statements
Use assert
statements to validate assumptions about your code and detect unexpected behavior.
def calculate_discount(price, discount):
assert discount >= 0 and discount <= 100, "Invalid discount percentage"
discounted_price = price - (price * discount / 100)
return discounted_price
5. Debugging Tools
Python offers built-in modules like pdb
and third-party tools like ipdb
for interactive debugging.
10 Code Examples for Debugging
Here are 10 common scenarios with debugging solutions:
- Syntax Error:
print("Hello, World!"
Solution: Add a closing parenthesis.
- Logical Error:
def calculate_area(radius):
return 2 * 3.14 * radius
Solution: Use 3.14159
for a more accurate approximation of π.
- Index Error:
numbers = [1, 2, 3]
print(numbers[3])
Solution: Use valid indices (0, 1, 2) for the list.
- Type Error:
result = "42" + 8
Solution: Convert the integer to a string or vice versa.
- Name Error:
print(variable_name)
Solution: Define variable_name
before using it.
- Attribute Error:
text = "Hello, World!"
print(text.length)
Solution: Use len(text)
to get the length.
- Value Error:
number = int("abc")
Solution: Convert a valid string representation of an integer.
- Key Error:
my_dict = {"key": "value"}
print(my_dict["missing_key"])
Solution: Ensure the key exists in the dictionary.
- Import Error:
import non_existent_module
Solution: Correct the module name or install the required package.
- Infinite Loop:
while True:
print("This loop runs forever!")
Solution: Add a condition to break out of the loop.
Conclusion
Python Debugging is an essential skill that every Python programmer should master. By understanding debugging concepts, leveraging the benefits, and practicing with code examples, you can become proficient in identifying and resolving errors in your Python code. Happy debugging!
FAQs for Python debugging
Q. What is debugging in Python?
A. Debugging is the process of identifying and resolving errors or issues in a Python program. It’s an essential step to ensure that the code runs as intended and produces the correct results.
Q. How do I start the Python debugger?
A. You can start the Python debugger by inserting import pdb; pdb.set_trace()
in your code where you want the debugger to stop and give you control. When the code execution reaches this line, it will pause, and you can interactively inspect variables, step through code, and more.
Q. What are some common Python debugging tools?
A. Some popular Python debugging tools include:
pdb
: The built-in Python debugger.ipdb
: An improved version of pdb with better command-line features.PyCharm
: An IDE that comes with a powerful debugger.Visual Studio Code
: Another IDE with debugging capabilities for Python.
Q. How do I set a breakpoint in Python?
A. In Python 3.7 and later, you can use the built-in breakpoint()
function to set a breakpoint. For earlier versions or if you’re using pdb
, you can insert import pdb; pdb.set_trace()
at the desired location in your code.
Q. What’s the difference between step
, next
, and continue
commands in the Python debugger?
A. In the Python debugger:
step
ors
: Executes the current line of code and stops at the first possible occasion (e.g., in a function that is called).next
orn
: Continues execution until the next line in the current function is reached.continue
orc
: Continues execution until the next breakpoint is encountered.
Q. How can I view the value of a variable while debugging?
A. While in the debugger, simply type the name of the variable and press enter. The debugger will display its current value. You can also use the print(variable_name)
command to display its value.
Q. How do I exit the Python debugger?
A. To exit the debugger, you can use the quit
or q
command. This will terminate the debugging session and the program.
Q. I’m getting a SyntaxError
. How do I debug it?
A. A SyntaxError
indicates that there’s a problem with the structure of your code. Common causes include missing parentheses, colons, or incorrect indentation. To debug, carefully review the error message and the line of code it points to. Ensure that the syntax of that line and the surrounding lines is correct.
Q. What is an exception in Python and how can I handle it?
A. An exception is an event that occurs during the execution of a program, signaling that an error has occurred. In Python, exceptions can be handled using try
and except
blocks. Code within the try
block is executed, and if an exception occurs, the code inside the corresponding except
block is executed.
Q. How can I see the call stack during debugging?
A. While in the Python debugger, you can use the where
or w
command to display the current position in the call stack. This shows you the sequence of function calls that led to the current point in the code.