In Python, a set is a built-in data type that stores an unordered collection of unique elements. Sets are similar to lists and tuples but differ in a few key aspects:
- Unordered: The elements in a set have no order.
- Unique: Sets do not allow duplicate elements.
- Mutable: You can add or remove elements from a set after it’s created, but the elements themselves must be immutable (e.g., numbers, strings, tuples).
Sets are a powerful data structure in Python that allow you to store unique elements with fast membership testing and efficient operations. Here are 10 python examples for sets:
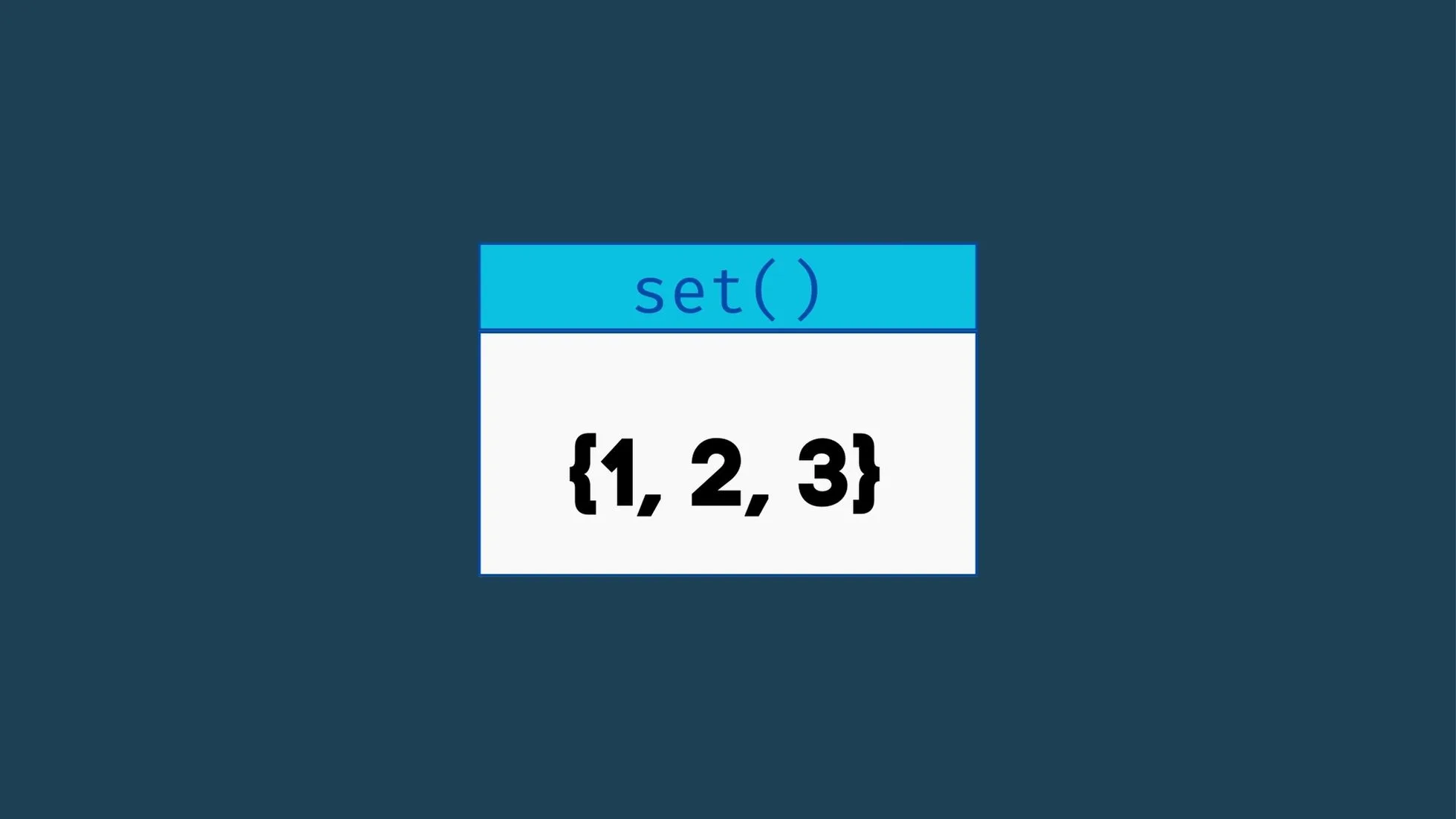
1. Creating a Set:
# Creating a set of unique elements
fruits = {'apple', 'banana', 'orange'}
2. Adding Elements to a Set:
# Adding elements to the set
fruits.add('grape')
fruits.add('apple') # Won't add since it's already in the set
3. Removing Elements from a Set:
# Removing an element from the set
fruits.remove('banana')
# Using discard to remove element (won't raise an error if element doesn't exist)
fruits.discard('kiwi')
4. Set Operations: Union, Intersection, Difference:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
intersection_set = set1.intersection(set2)
difference_set = set1.difference(set2) # Elements in set1 but not in set2
5. Checking Subset and Superset:
subset_check = set1.issubset(set2)
superset_check = set1.issuperset(set2)
6. Removing Duplicate Elements from a List:
num_list = [1, 2, 2, 3, 4, 4, 5]
unique_nums = set(num_list) # Convert list to set to remove duplicates
7. Finding Common Elements in Multiple Sets:
set3 = {2, 4, 6}
common_elements = set1.intersection(set2, set3)
8. Removing Duplicates from a String:
sentence = "hello world hello"
unique_words = set(sentence.split()) # Split the sentence into words and convert to set
9. Checking Disjoint Sets:
disjoint_check = set1.isdisjoint(set3) # Returns True if no common elements
10. Set Comprehensions:
squared_set = {x**2 for x in range(1, 6)} # Creating a set of squared numbers