Control flow is a fundamental concept in programming that allows you to control how your code is executed. In Python, control flow methods are managed through conditional statements, loops, and function calls. In this blog post, we’ll explore these concepts in detail with examples.
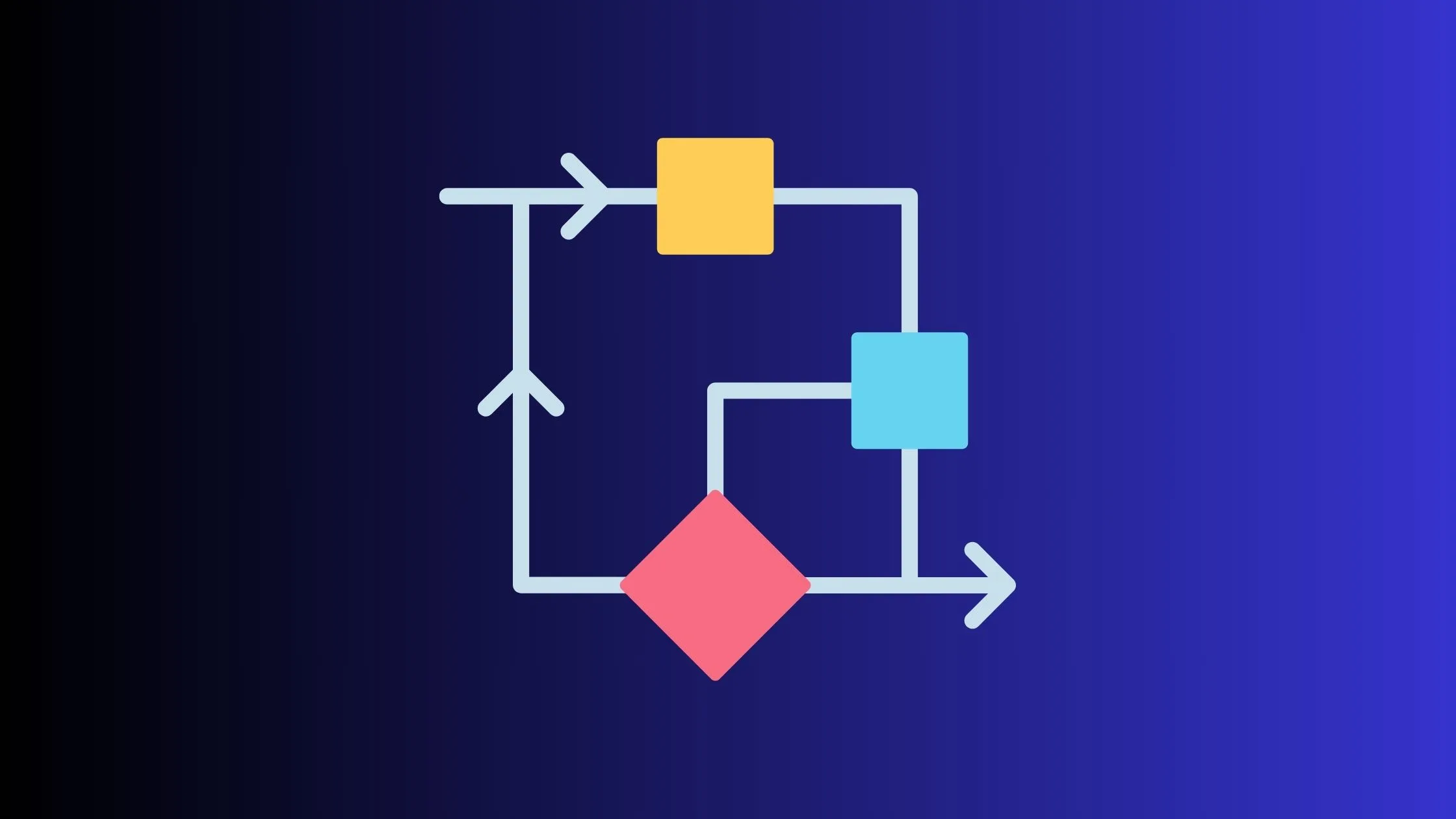
Table of Contents
Conditional Statements
1. If Statement
The if
statement is used to test a condition and execute a block of code if the condition is true.
x = 10
if x > 5:
print("x is greater than 5")
2. If-Else Statement
The if-else
statement allows you to execute one block of code if a condition is true and another block if it’s false.
x = 3
if x > 5:
print("x is greater than 5")
else:
print("x is not greater than 5")
3. If-Elif-Else Statement
The if-elif-else
statement lets you test multiple conditions.
x = 10
if x > 20:
print("x is greater than 20")
elif x > 10:
print("x is greater than 10 but less than 20")
else:
print("x is less than or equal to 10")
Loops
1. For Loop
The for
loop is used to iterate over a sequence (like a list or tuple).
for i in range(5):
print(i)
2. While Loop
The while
loop continues to execute a block of code as long as a condition is true.
x = 0
while x < 5:
print(x)
x += 1
Certainly! The pass
and break
statements in Python are used within loops and conditional statements, and they serve different purposes. Let’s explore both of them with code examples.
Pass Statement
The pass
statement is a no-operation statement that serves as a placeholder. It’s used when a statement is syntactically required but you don’t want to execute any code.
Example:
for i in range(5):
if i == 3:
pass
print(i)
In this example, the pass
statement does nothing when i
is 3, and the loop continues to the next iteration. All numbers from 0 to 4 will be printed.
Break Statement
The break
statement is used to exit a loop prematurely when a certain condition is met.
Example:
for i in range(5):
if i == 3:
break
print(i)
In this example, the loop will exit when i
is 3, so only the numbers 0, 1, and 2 will be printed.
Comparison Between Pass and Break
- Pass: It’s like a placeholder and does nothing. The loop continues to the next iteration.
- Break: It exits the loop entirely, and the code following the loop (if any) continues to execute.
Using Both in a Loop
Here’s an example that demonstrates the use of both pass
and break
in a loop:
for i in range(5):
if i == 1:
pass
elif i == 3:
break
print(i)
In this code:
- When
i
is 1, thepass
statement is executed, and the loop continues to the next iteration. - When
i
is 3, thebreak
statement is executed, and the loop exits.
The output will be:
0
1
2
Understanding the pass
and break
statements and how to use them appropriately can help you write more concise and controlled code in Python.
Continue Statement
The continue
statement is used to skip the current iteration of a loop and continue with the next iteration. When a continue
statement is encountered, the remaining code inside the loop for that iteration is skipped, and the loop’s controlling expression (such as a while
or for
statement) is re-evaluated to determine whether the loop will execute again.
Example:
for i in range(5):
if i == 2:
continue
print(i) # Output: 0 1 3 4</code>
Here, when i
is 2, the continue
statement is executed, and the print(i)
statement is skipped for that iteration, so the value 2 is not printed.
Functions
Functions allow you to define a block of code that can be called by its name elsewhere in your code.
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
Conclusion
Python’s control flow structures are powerful tools that allow you to write flexible and efficient code. By understanding how to use conditional statements, loops, and functions, you can create programs that respond to different conditions and repeat actions as needed.
Whether you’re a beginner or an experienced programmer, mastering control flow in Python will help you write more robust and readable code.