Introduction:
In a retail store, keeping track of the inventory is crucial for maintaining optimal stock levels and improving customer satisfaction. Python list can be effectively used to manage inventory items, their prices, and quantities. Lists are dynamic, easy to manipulate, and can be used to perform a variety of operations like sorting, appending, slicing, and more. Following is an example of simple Inventory Management system.
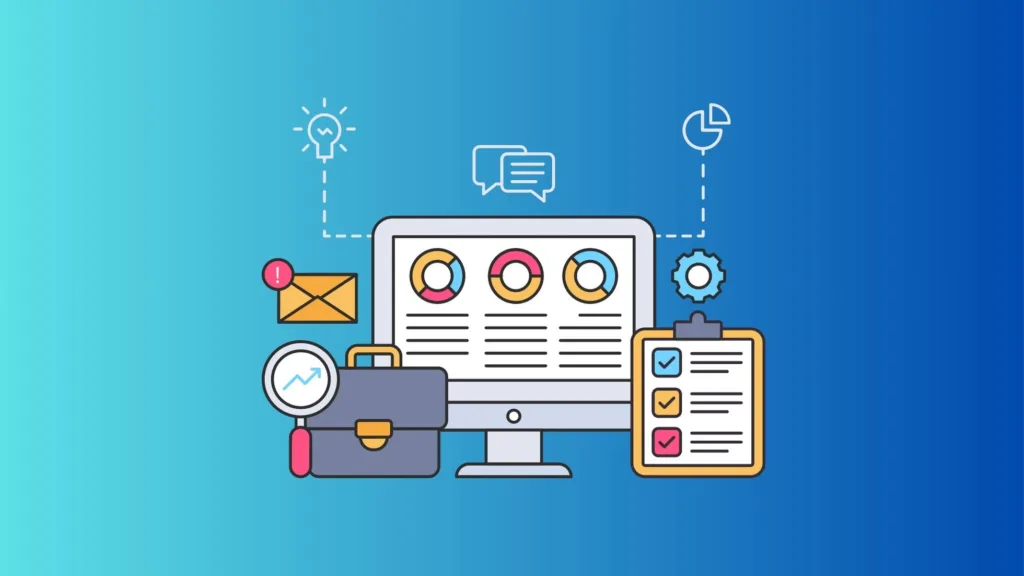
Code Example for Inventory Management using Python List:
This code manages an inventory system for various items. Here’s a summary of what the code does:
Initialization:
- Three lists are initialized:
inventory_items
containing names of inventory items.item_prices
containing prices corresponding to the items.item_quantities
containing quantities of each item in stock.
Display Inventory Function:
display_inventory
is a function that prints the current inventory in a tabular format, including item names, prices, and quantities. It iterates through the lists and prints each item’s details.
Add Item Function:
add_item
is a function that allows adding a new item to the inventory. It takes the item name, price, and quantity as arguments and appends them to the respective lists.
Remove Item Function:
remove_item
is a function for removing an item from the inventory. It identifies the index of the item in the lists and removes it from all three lists.
Update Quantity Function:
update_quantity
is a function that updates the quantity of a specific item in the inventory. It finds the index of the item and updates the corresponding quantity.
Displaying Initial Inventory using Python List:
- The script starts by displaying the current inventory using the
display_inventory
function.
# Initializing lists for inventory items, their prices and quantities
inventory_items = ["Toothpaste", "Shampoo", "Soap", "Toothbrush", "Deodorant"]
item_prices = [2.5, 5.0, 1.2, 0.8, 3.0]
item_quantities = [100, 50, 200, 150, 70]
# Function to display inventory
def display_inventory(items, prices, quantities):
print("Current Inventory:")
print("Item\t\tPrice\t\tQuantity")
for i in range(len(items)):
print(f"{items[i]}\t\t{prices[i]}\t\t{quantities[i]}")
# Function to add a new item to the inventory
def add_item(item, price, quantity):
inventory_items.append(item)
item_prices.append(price)
item_quantities.append(quantity)
# Function to remove an item from the inventory
def remove_item(item):
index = inventory_items.index(item)
inventory_items.pop(index)
item_prices.pop(index)
item_quantities.pop(index)
# Function to update quantity of an item in the inventory
def update_quantity(item, new_quantity):
index = inventory_items.index(item)
item_quantities[index] = new_quantity
# Displaying current inventory
display_inventory(inventory_items, item_prices, item_quantities)
# Adding a new item
add_item("Hand Sanitizer", 2.0, 100)
print("\nAfter adding a new item:")
display_inventory(inventory_items, item_prices, item_quantities)
# Removing an item
remove_item("Toothbrush")
print("\nAfter removing an item:")
display_inventory(inventory_items, item_prices, item_quantities)
# Updating the quantity of an item
update_quantity("Soap", 250)
print("\nAfter updating quantity:")
display_inventory(inventory_items, item_prices, item_quantities)
Output:
Current Inventory:
Item Price Quantity
Toothpaste 2.5 100
Shampoo 5.0 50
Soap 1.2 200
Toothbrush 0.8 150
Deodorant 3.0 70
After adding a new item:
Current Inventory:
Item Price Quantity
Toothpaste 2.5 100
Shampoo 5.0 50
Soap 1.2 200
Toothbrush 0.8 150
Deodorant 3.0 70
Hand Sanitizer 2.0 100
After removing an item:
Current Inventory:
Item Price Quantity
Toothpaste 2.5 100
Shampoo 5.0 50
Soap 1.2 200
Deodorant 3.0 70
Hand Sanitizer 2.0 100
After updating quantity:
Current Inventory:
Item Price Quantity
Toothpaste 2.5 100
Shampoo 5.0 50
Soap 1.2 250
Deodorant 3.0 70
Hand Sanitizer 2.0 100
In this example, Python lists are used to manage and manipulate the inventory items, their prices, and quantities in a retail store. The example showcases basic CRUD operations (Create, Read, Update, Delete) on the inventory.