Functional programming in Python is an important topic that often comes up in interviews. Below are some popular interview questions along with their answers and code examples.
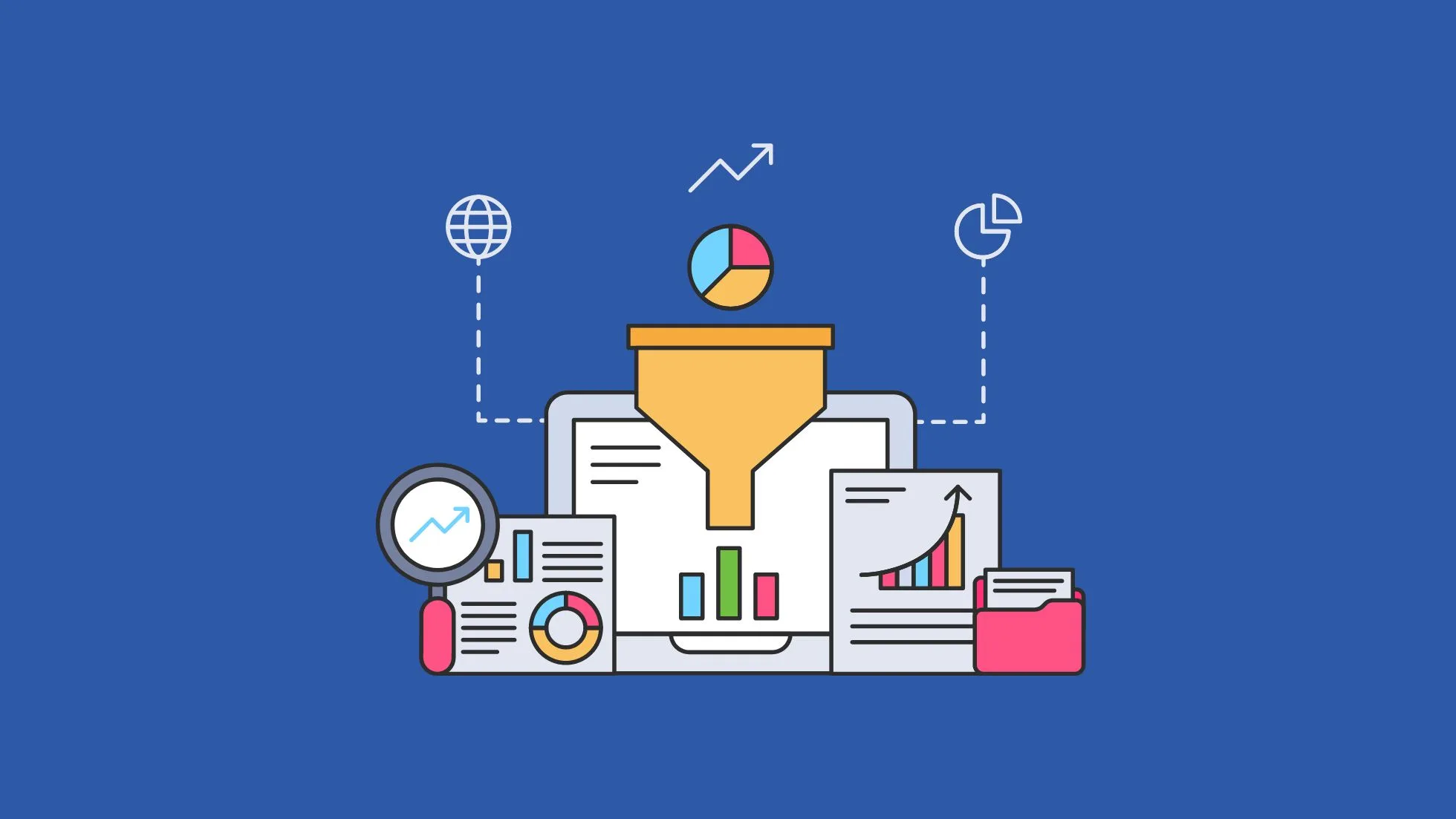
Question 1: What is functional programming?
Answer: Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state or mutable data.
Question 2: What are pure functions?
Answer: A pure function is a function where the output is determined only by its input, without observable side effects.
# Pure Function Example
def square(x):
return x * x
Question 3: Explain first-class functions in Python.
Answer: In Python, functions are first-class citizens, meaning they can be passed around as arguments to other functions, returned as values from other functions, and assigned to variables.
# First-Class Function Example
def greet(name):
return f"Hello, {name}"
say_hello = greet
print(say_hello("John"))
Question 4: What are higher-order functions?
Answer: Higher-order functions are functions that take one or more functions as arguments or return a function as a result.
# Higher-order Function Example
def apply(func, value):
return func(value)
print(apply(square, 5))
Question 5: What is a lambda function?
Answer: A lambda function is a small anonymous function defined with the lambda
keyword.
# Lambda Function Example
double = lambda x: x * 2
print(double(4))
Question 6: How to use map()
in Python?
Answer: map()
applies a function to all items in an input list.
# map() Example
result = map(square, [1, 2, 3, 4])
print(list(result))
Question 7: Explain filter()
in Python.
Answer: filter()
filters the elements of a list based on a function, returning a new list with elements that evaluate to True
.
# filter() Example
result = filter(lambda x: x % 2 == 0, [1, 2, 3, 4])
print(list(result))
Question 8: What is reduce()
in Python?
Answer: reduce()
applies a rolling computation to sequential pairs of values in a list, reducing the list to a single value.
from functools import reduce
# reduce() Example
result = reduce(lambda x, y: x*y, [1, 2, 3, 4])
print(result)
Question 9: What are closures in Python?
Answer: A closure is a function object that has access to variables in its enclosing lexical scope.
# Closure Example
def outer(x):
def inner(y):
return x + y
return inner
closure = outer(10)
print(closure(5))
Question 10: What is currying in Python?
Answer: Currying is the technique of breaking down a function that takes multiple arguments into a series of functions that each take a single argument.
# Currying Example
def multiply(x):
def wrapper(y):
return x * y
return wrapper
double = multiply(2)
print(double(4))
Question 11: Explain recursion in functional programming.
Answer: Recursion is a technique where a function calls itself.
# Recursion Example
def factorial(n):
if n == 1:
return 1
return n * factorial(n-1)
Question 12: What is immutability?
Answer: Immutability refers to the idea that data should not be changed once created.
# Using tuple for immutability
x = (1, 2, 3)
Question 13: How do you use the zip
function?
Answer: zip
is used to combine multiple iterables element-wise.
# zip() Example
result = zip([1, 2, 3], ['a', 'b', 'c'])
print(list(result))
Question 14: What are decorators in Python?
Answer: A decorator is a design pattern that allows you to add new functionality to an existing object without modifying its structure. In Python, decorators dynamically alter the functionality of a function or class.
# Decorator Example
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
Question 15: What are Python Generators?
Answer: Generators are iterators that generate values on-the-fly and do not store them in memory.
# Generator Example
def count_up_to(n):
count = 1
while count <= n:
yield count
count += 1
Question 16: How to create an infinite sequence in Python?
Answer: You can create an infinite sequence using generators.
# Infinite sequence generator
def infinite_sequence():
num = 0
while True:
yield num
num += 1
Question 17: What is the role of __iter__()
and __next__()
in Python?
Answer: __iter__()
returns the iterator object and __next__()
returns the next value from the iterator.
# __iter__() and __next__() Example
class MyRange:
def __init__(self, n):
self.n = n
self.i = 0
def __iter__(self):
return self
def __next__(self):
if self.i < self.n:
value = self.i
self.i += 1
return value
else:
raise StopIteration
Question 18: Explain the concept of “Lazy Evaluation.”
Answer: Lazy evaluation means that the evaluation of an expression is delayed until its value is actually needed. Generators in Python are an example of lazy evaluation.
# Lazy evaluation with generator
lazy_eval = (x*x for x in range(1, 11))
Question 19: How is functools.partial
used in Python?
Answer: functools.partial
allows you to fix a certain number of arguments of a function and generate a new function.
from functools import partial
# functools.partial Example
def multiply(x, y):
return x * y
double = partial(multiply, 2)
print(double(4))
Question 20: How is functional programming different from object-oriented programming?
Answer: In functional programming, the focus is on what you are computing, whereas in object-oriented programming, the focus is on the objects that you are manipulating.