Data compression in Python is an essential technique that reduces the storage space needed for data files and allows for faster data transmission. It plays a vital role in optimizing storage, network bandwidth, and computing resources. In Python, you have several libraries at your disposal to perform data compression, making the process easy and efficient. This blog post will explore different methods for data compression in Python using built-in libraries and demonstrate examples of each method.
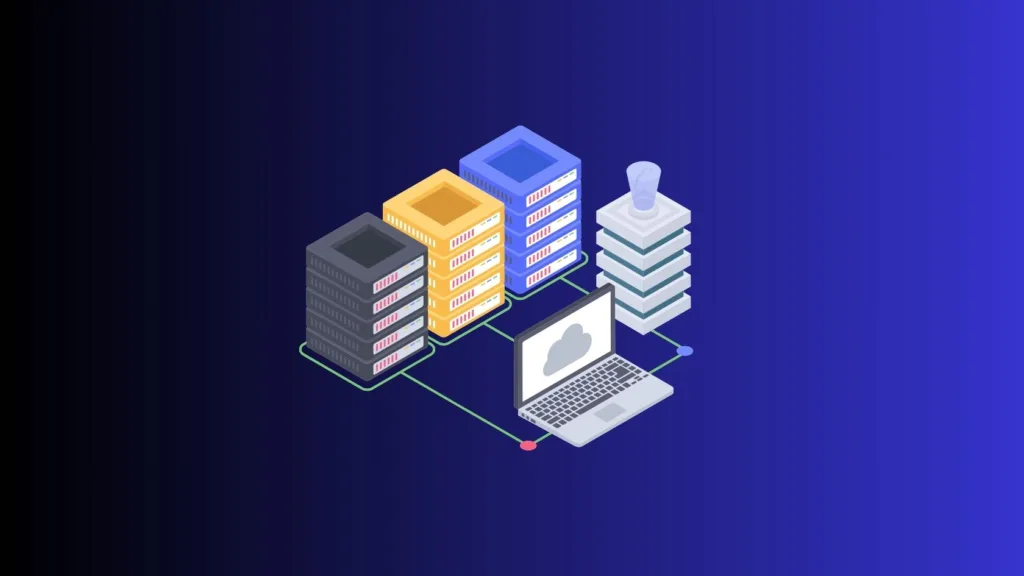
1. Introduction to Data Compression in Python
Data compression involves encoding information using fewer bits than the original representation. There are two main types of compression: lossless and lossy. Lossless compression ensures that the original data can be fully restored, whereas lossy compression may lose some information in the process.
2. Compression Techniques
a. Zip
The zipfile
module in Python allows you to work with ZIP archives. Here’s an example of compressing a file:
import zipfile
with zipfile.ZipFile('example.zip', 'w') as myzip:
myzip.write('example.txt')
b. Gzip
The gzip
module in Python provides a simple way to work with GZIP files. Here’s an example:
import gzip
with gzip.open('example.txt.gz', 'wt') as f:
f.write('Hello, World!')
c. Bzip2
The bz2
module in Python enables compression using the Bzip2 algorithm:
import bz2
content = b"Hello, World!"
compressed_data = bz2.compress(content)
d. LZMA
The lzma
module in Python is used for LZMA compression:
import lzma
content = b"Hello, World!"
compressed_data = lzma.compress(content)
3. Working with Archive Files
The shutil
module in Python allows you to work with various archive formats, including creating and extracting tar and zip files. Here’s an example:
import shutil
shutil.make_archive('example', 'zip', '.', 'example_dir')
4. Custom Compression Algorithms
For more specific needs, you may develop your custom compression algorithms using Python’s robust set of tools and libraries. This will require a deeper understanding of compression techniques and algorithms.
5. Conclusion
Data compression is a vital process in today’s data-driven world. Python provides a wide array of libraries and modules to make data compression simple and effective. Whether you are looking to work with standard compression formats or develop custom solutions, Python offers the tools and flexibility you need.
Always consider the trade-offs between compression level, speed, and compatibility with your specific use case to choose the most appropriate compression method.
6. FAQs for Data Compression in Python
Q. What is data compression in Python?
A. Data compression in Python refers to the process of reducing the size of data, making it more efficient for storage or transmission. Python offers various libraries and modules, such as zlib
, gzip
, and bzip2
, to facilitate this process.
Q. Why would I want to use data compression in my Python application?
A. There are several reasons:
- To save storage space, especially when dealing with large datasets.
- To speed up data transmission, especially over slow or limited networks.
- To obfuscate data, making it harder to read without the proper decompression key or method.
Q. How can I compress data using the zlib
module in Python?
A. You can use the compress
method from the zlib
module. Here’s a basic example:
import zlib
data = b"Your data here"
compressed_data = zlib.compress(data)
Q. How do I decompress data that has been compressed using zlib
?
A. You can use the decompress
method from the zlib
module:
import zlib
decompressed_data = zlib.decompress(compressed_data)
Q. Are there any drawbacks to compressing data?
A. While compression can save storage space and speed up transmission, it can also introduce some overhead during the compression and decompression processes. This might not be suitable for real-time applications where speed is critical. Additionally, if the compression algorithm introduces any errors, the original data might be lost or corrupted.
Q. Can I compress strings or text files in Python?
A. Absolutely! Libraries like gzip
are specifically designed for compressing text. For strings, you can first convert the string to bytes using str.encode()
and then compress it.
Q. What’s the difference between gzip
and zlib
?
A. Both are compression libraries, but there are some differences:
gzip
is specifically designed for compressing files and is commonly used in UNIX systems for file compression.zlib
is a more general-purpose library and can be used for compressing any data in memory. It doesn’t include file headers, making it more suitable for compressing small blocks of data.
Q. How secure is data compression? Can it be used for encryption?
A. Data compression is not inherently secure and should not be confused with encryption. While compressed data might look obfuscated, it can be decompressed by anyone with the right tool or knowledge. If you need to secure your data, it’s recommended to use encryption techniques in conjunction with compression.
Q. Are there any third-party Python libraries for data compression?
A. Yes, there are several third-party libraries like lzma
, lz4
, and snappy
that offer different compression algorithms and might be more suitable for specific use cases or offer better compression rates.