Defining Python functions is a fundamental aspect of Python programming. However, there are common pitfalls and mistakes that developers, especially beginners, might encounter. This blog post will explore some of these common mistakes, offering explanations and solutions to help you write more robust and error-free functions.
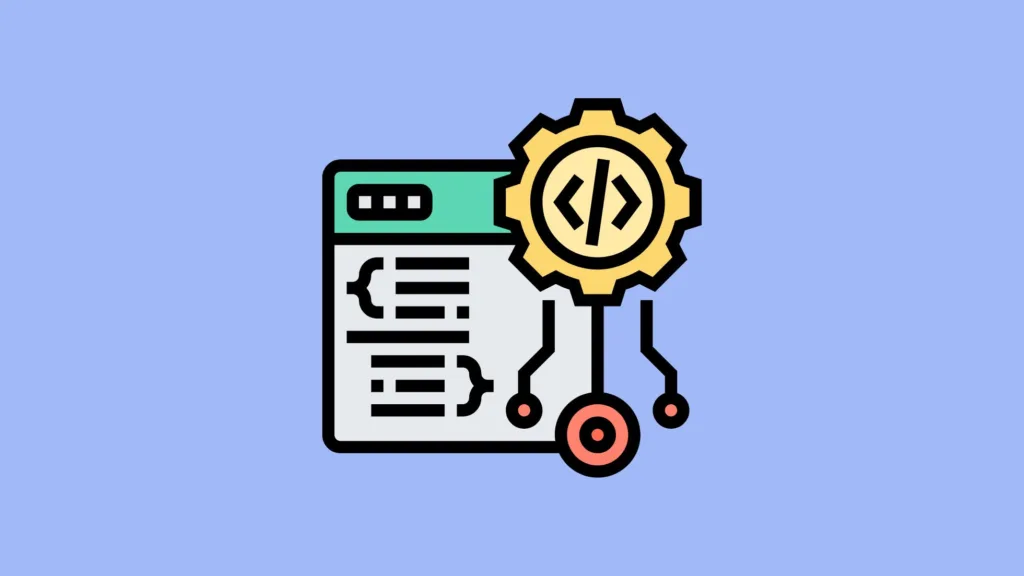
Table of Contents
- Mistakes in Defining Python Functions
- Syntax Errors
- Parameter Conflicts
- Variable Scope Confusion
- Return Statement Mistakes
- Mutability Issues
- Conclusion
Mistakes in Defining Python Functions
Syntax Errors
Missing Colon
Forgetting the colon :
after the function definition leads to a syntax error.
Example:
def my_function() # Missing colon
print("Hello, World!")
Solution:
def my_function(): # Correct
print("Hello, World!")
Indentation Errors
Incorrect indentation inside the function can cause syntax errors.
Example:
def my_function():
print("Hello, World!") # Incorrect indentation
Solution:
def my_function():
print("Hello, World!") # Correct indentation
Parameter Conflicts
Default Arguments After Non-Default
Placing default arguments before non-default arguments in the function definition leads to a syntax error.
Example:
def display(name="Alice", age): # Incorrect order
print(name, age)
Solution:
def display(age, name="Alice"): # Correct order
print(name, age)
Variable Scope Confusion
Using Global Variables Without Declaration
Accessing or modifying a global variable inside a function without declaring it as global can lead to unexpected behavior.
Example:
x = 10
def update_x():
x = 5 # This creates a local variable, not modifying the global x
update_x()
print(x) # Outputs 10
Solution:
x = 10
def update_x():
global x # Declare x as global
x = 5
update_x()
print(x) # Outputs 5
Return Statement Mistakes
Missing Return Statement
Forgetting to include a return statement in a function expected to return a value leads to returning None
.
Example:
def add(a, b):
result = a + b # Missing return statement
print(add(2, 3)) # Outputs None
Solution:
def add(a, b):
return a + b # Correct
print(add(2, 3)) # Outputs 5
Mutability Issues
Modifying Mutable Default Arguments
Using mutable default arguments like lists or dictionaries can lead to unexpected behavior.
Example:
def append_item(item, lst=[]): # Using mutable default argument
lst.append(item)
return lst
print(append_item(1)) # Outputs [1]
print(append_item(2)) # Outputs [1, 2], not [2]
Solution:
def append_item(item, lst=None):
if lst is None:
lst = []
lst.append(item)
return lst
Conclusion
Defining Python functions is relatively straightforward, but it’s easy to fall into some common traps. Being aware of these common mistakes and understanding how to avoid them can make your code more robust and maintainable. Always remember to follow the correct syntax, be cautious with parameter handling, understand variable scope, use return statements appropriately, and be mindful of mutability when working with functions in Python.